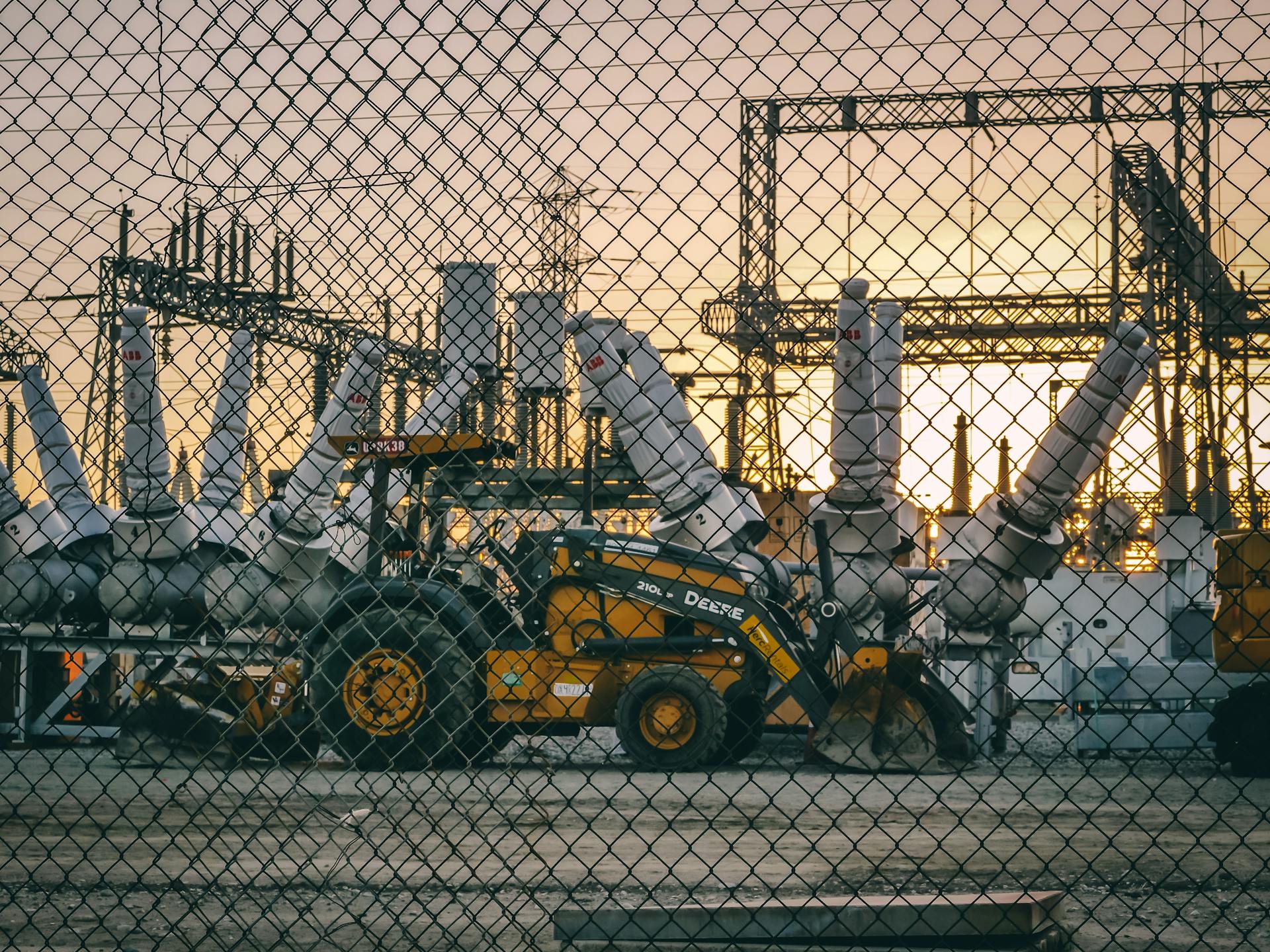
Asynchronous generators in Python are a powerful tool for handling complex tasks, allowing you to write efficient and readable code.
They work by producing a series of values on demand, rather than all at once, which makes them ideal for handling large datasets or infinite sequences.
This approach can significantly improve performance, as it avoids the need for unnecessary memory allocation and copying of data.
In Python, you can create an asynchronous generator using the async def syntax and the yield keyword, which suspends the execution of the function until the next value is requested.
A different take: Asynchronous Generator
Advantages and Disadvantages of Asynchronous Generators
The asynchronous generator, also known as an induction generator, has several advantages that make it a popular choice for power generation.
Its robust construction requires less maintenance, which means you'll spend less time and money keeping it running smoothly.
The induction generator is also a cost-effective option, as it's less expensive than other types of generators.
For your interest: Asynchronous Motor as Generator
One of the most convenient features of an induction generator is that it can be used as a squirrel cage, making it a versatile option.
The size of an induction generator is relatively small compared to its output power, making it a great choice for smaller power generation needs.
You don't need to worry about synchronizing the generator with the supply line for it to operate, which simplifies the setup process.
An induction generator requires fewer auxiliary equipment, which reduces the overall complexity of the system.
In the event of a short-circuit fault, the induction generator has a self-protective feature that stops generation, preventing further damage.
Here are some of the key advantages of an induction generator:
- Robust construction
- Less expensive
- Can be used as a squirrel cage
- Small size per kW output power
- No synchronism with supply line required
- Less number of auxiliary equipment
- Self-protective feature
- Runs in parallel without hunting
Python Asynchronous Generator Syntax and Semantics
Python asynchronous generators are defined using the async def keyword, and the function body should contain the yield statement. They were introduced in Python 3.6 with the PEP 525 proposal.
To create an asynchronous generator, you need to define a coroutine function that utilizes the yield expression. The async def keyword is used to declare the function, and the yield statement is used to produce values.
A unique perspective: Asynchronous Generator vs Synchronous Generator
Native async generators don't support yield from, but our library does. You can use yield from inside an @async_generator function, and it can be any kind of async iterator, including native async generators.
The return type of an async generator is an asynchronous generator object, which implements both __aiter__ and __anext__ methods. This allows you to iterate over it asynchronously using an async for loop.
An async generator is not a regular iterable, meaning you can't use a traditional for loop due to its asynchronous nature. Instead, async generators are asynchronous iterables that must be processed using an async for loop.
The async for loop was introduced alongside async generators in Python 3.6 and makes it straightforward to iterate over the yielded values from the async generator.
Understanding Asynchronous Generators
Asynchronous generators are a powerful tool in Python that allow you to create iterators that can suspend and resume their execution.
They can be used to create complex iterators that can handle multiple tasks and operations.
A different take: Generators Create Electrical Energy.
An asynchronous generator can be defined using the async def keyword, and it can use the yield keyword to produce a value.
In the case of an asynchronous generator, the yield keyword is used to produce an awaitable value that can be awaited by the main coroutine.
This is useful for creating iterators that can handle multiple tasks and operations, such as iterating over a list of tasks and awaiting each task's completion.
To step an asynchronous generator one iteration, you can use the anext() function, which returns an awaitable that can be awaited to execute one iteration of the generator.
The anext() function is useful for understanding the coroutine nature of asynchronous generators, which can suspend and await other coroutines and tasks.
Running the example with anext() first creates the main coroutine and uses it as the entry point into the asyncio program, then it creates an instance of the asynchronous generator and calls the anext() function on it.
The anext() function returns an awaitable that if awaited will execute one iteration of the generator to the first yield statement, and a value is returned.
The main coroutine resumes and retrieves the yielded value and reports it, highlighting what is happening when an asynchronous generator is executed.
Asynchronous generators can be traversed using the async for expression, which automatically awaits each awaitable returned from the generator.
This is perhaps the most common usage pattern for asynchronous generators, and it's useful for iterating over a complex iterator and handling multiple tasks and operations.
The async for expression uses the anext() function to step the generator one iteration at a time, and it retrieves the yielded value and makes it available within the loop body.
This process is repeated until the generator is exhausted, and it's useful for creating complex iterators that can handle multiple tasks and operations.
Asynchronous generators can also be used with asynchronous list comprehensions, which can collect the yielded values into a list that can be reported.
This is useful for creating complex iterators that can handle multiple tasks and operations, and it's a powerful tool in Python for creating iterators that can suspend and resume their execution.
Best Practices and Takeaways
Asynchronous generators in Python can be a game-changer for your coding workflow.
You now know how to create and use asynchronous generators in Python, which is a huge accomplishment.
Working with Iterators and Context Managers
Working with iterators and context managers is a breeze with asynchronous generators. You can use an async for loop to iterate through an asynchronous generator and process its items concurrently.
The async for loop is a powerful tool for working with asynchronous generators. To use it, simply use the async for keyword in your code.
Async context managers are also a great way to handle resources like files and sockets. You can create your own async context managers using the @asynccontextmanager decorator, which is available in the contextlib library.
The contextlib library is a useful tool for context and resource management, and it integrates well with asyncio. With async context managers, you can ensure that resources are properly cleaned up after use.
To create an async generator, you need to define a coroutine function that uses the yield expression. This will produce values from the generator. You can use the async def keyword to declare the function and include the yield statement to produce values.
The aclosing async context manager is a convenient way to explicitly call aclose on an asynchronous generator. It acts just like the closing context manager included in the stdlib contextlib module, but awaits obj.aclose() instead of obj.close().
Discover more: Does a Generator Produce Ac or Dc
Python Programming and Asynchronous Generators
Python 3.6 introduced asynchronous generators with the PEP 525 proposal, making it easier to handle asynchronous tasks efficiently.
Asynchronous generators use the async def and yield keywords, which enable developers to create asynchronous tasks.
The async def keyword is used to declare the function, and the yield statement is used to produce values.
You can define a coroutine function that utilizes the yield expression to create an asynchronous generator.
The async def keyword is a must when creating an asynchronous generator in Python.
Asynchronous generators can be used to handle tasks more efficiently, making them a valuable tool in Python programming.
Sources
- https://www.tutorialspoint.com/induction-generator-asynchronous-generator
- https://peps.python.org/pep-0525/
- https://superfastpython.com/asynchronous-generators-in-python/
- https://async-generator.readthedocs.io/en/latest/reference.html
- https://blog.finxter.com/python-async-generator-mastering-asyncio-in-modern-applications/
Featured Images: pexels.com